Spring framework Interview Questions
Author: Vivek Prasad
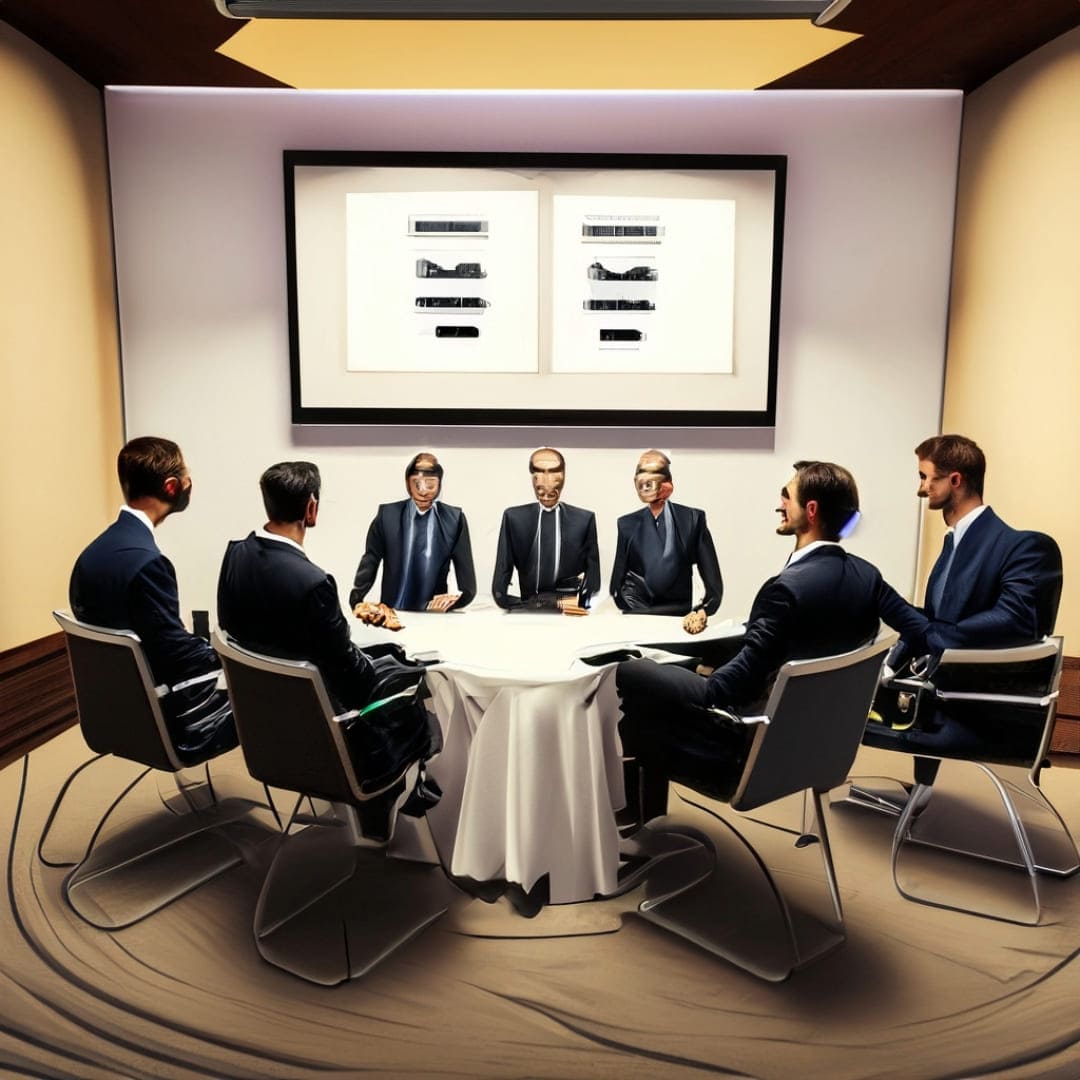
Q1) What are types of beans in a spring framework?
In the context of the Spring Framework, a "bean" refers to a Java object that is managed by the Spring container. Spring beans are used to represent various components in an application, and they are configured and managed by the Spring framework.
There are several types of beans in Spring, each serving a different purpose:
In the context of the Spring Framework, a "bean" refers to a Java object that is managed by the Spring container. Spring beans are used to represent various components in an application, and they are configured and managed by the Spring framework.
There are several types of beans in Spring, each serving a different purpose:
Singleton Beans:
Singleton beans are the most common type of beans in Spring. A single instance of the bean is created, and it is shared across the entire application. By default, all Spring beans are singleton unless specified otherwise.
• Singleton beans are the most common type of beans in Spring.
• A single instance of the bean is created, and it is shared across the entire application.
• By default, all Spring beans are singleton unless specified otherwise.
• A single instance of the bean is created, and it is shared across the entire application.
• By default, all Spring beans are singleton unless specified otherwise.
Prototype Beans:
• Prototype beans are different from singleton beans in which a new instance of the bean is created each time it is requested from the Spring container.
• Prototype beans are not shared among different parts of the application.
• Prototype beans are not shared among different parts of the application.
Request Scope Beans:
• Request scope beans are created for each HTTP request when used in a web application.
• They exist only for the duration of a single HTTP request/response cycle and are discarded afterward.
• They exist only for the duration of a single HTTP request/response cycle and are discarded afterward.
Session Scope Beans:
• Session scope beans are created once for each user session in a web application.
• They exist as long as the user's session is active and are discarded when the session ends.
• They exist as long as the user's session is active and are discarded when the session ends.
Application Scope Beans:
• Application scope beans are created once for the entire lifetime of a web application.
• They are shared among all users and all requests within the application.
• They are shared among all users and all requests within the application.
Custom Scope Beans:
• Spring allows you to define custom scopes by implementing the org.springframework.beans.factory.config.Scope interface.
• This allows you to create beans with custom lifecycles tailored to your application's needs.
• This allows you to create beans with custom lifecycles tailored to your application's needs.
Singleton Beans with Prototype Dependencies:
• In some cases, you may want a singleton bean to depend on a prototype-scoped bean. This can be achieved by using method injection or by using the lookup-method element in XML configuration.
Lazy Initialization:
• Spring allows you to specify lazy initialization for beans. Lazy initialization means that a bean is created only when it is first requested, rather than at application startup.
Inner Beans:
• Inner beans are beans that are defined within the scope of another bean and are typically used when a bean is only used by the enclosing bean and does not need to be exposed globally.
Factory Beans:
• Factory beans are used to create and configure complex objects in a controlled manner. They are often used when the process of creating a bean is non-trivial.
Bean References:
• Sometimes, beans need to reference other beans. You can use the ref attribute or @Autowired annotation to inject references to other beans.
Null Beans:
• You can define a bean to represent a null object, useful in cases where you want to provide a default value or a placeholder. Spring's flexibility in managing different types of beans allows you to create complex and adaptable application structures while promoting good design practices such as dependency injection and inversion of control (IoC). The choice of which type of bean to use depends on the specific requirements and scope of your application components.
Q2) What is autowiring in Spring
Autowiring is a feature in the Spring Framework that allows you to automatically inject (wire) dependencies into Spring beans without explicitly specifying the dependencies in the bean configuration file (XML) or using annotations. Autowiring simplifies the process of managing dependencies and promotes loose coupling between components in a Spring application.
In Spring, you can use autowiring in several ways to inject dependencies:
No Autowiring (Default):
• If you do not specify autowiring explicitly, Spring will default to "no autowiring," which means you must manually configure dependencies for each bean in the XML configuration or using annotations like @Autowired.
Autowiring by Type (autowire="byType" or @Autowired):
• In this mode, Spring looks for a bean of the same data type as the property or constructor argument and injects it. If a unique bean of that type is found, it's injected; otherwise, it results in an error.
Example
@Autowired
private MyDependency myDependency;
<bean id="myService" class="com.example.MyService" autowire="byType"/>
Autowiring by Name (autowire="byName"):
• With autowiring by name, Spring looks for a bean with the same name as the property or constructor argument and injects it.
Example using XML configuration:
<bean id="myService" class="com.example.MyService" autowire="byName"/>
Autowiring by Qualifier (@Autowired with @Qualifier):
• This mode is used in combination with @Autowired to specify a particular bean by its name when there are multiple beans of the same type.
Example using annotations:
@Autowired
@Qualifier("specificBean")
private MyDependency myDependency;
Autowiring by Constructor (autowire="constructor"):
• With this mode, Spring looks for a constructor in the bean class and attempts to automatically satisfy its dependencies by matching constructor argument types with available beans.
Example using XML configuration:
<bean id="myService" class="com.example.MyService" autowire="constructor"/>
Autowiring can simplify configuration and reduce the amount of boilerplate code required for dependency injection, especially in large Spring applications with many beans and dependencies. However, it's essential to use autowiring judiciously and understand its implications, as it may not always provide the desired behavior, particularly in complex scenarios with multiple beans of the same type or circular dependencies.
Q3) Describes the few most used annotations in Spring
Spring Framework provides a variety of annotations that are commonly used to configure and manage different aspects of a Spring application. Here are some of the most commonly used annotations in Spring:
@Component and Derived Annotations:
• @Component is the base annotation for declaring a class as a Spring bean.
• Derived annotations like @Service, @Repository, and @Controller are specialized forms of @Component that provide additional behavior. These annotations help categorize beans and are often used for specific layers of an application.
• Derived annotations like @Service, @Repository, and @Controller are specialized forms of @Component that provide additional behavior. These annotations help categorize beans and are often used for specific layers of an application.
@Autowired:
• @Autowired is used for automatic dependency injection. It allows Spring to inject dependencies into fields, constructors, or setter methods of a bean.
@Configuration:
• @Configuration is used to define Java-based configuration classes. These classes can contain bean definitions using @Bean methods.
@Bean:
• @Bean is used to define a method as a producer of a bean. The return value of the method is registered as a Spring bean.
@Value:
• @Value is used to inject values from property files, environment variables, or other sources into bean fields or constructor parameters.
@Qualifier:
• @Qualifier is used in combination with @Autowired to specify which bean should be injected when multiple beans of the same type are available.
@Primary:
• @Primary is used to indicate that a particular bean should be considered as the primary candidate when multiple beans of the same type are available for autowiring.
@Scope:
• @Scope is used to define the scope of a bean, such as singleton, prototype, request, session, etc.
@PostConstruct and @PreDestroy:
• @PostConstruct is used to specify a method that should be executed after bean initialization.
• @PreDestroy is used to specify a method that should be executed before a bean is destroyed.
• @PreDestroy is used to specify a method that should be executed before a bean is destroyed.
@RequestMapping (in Spring MVC):
• @RequestMapping is used to map HTTP requests to controller methods in Spring MVC applications.
@RestController (in Spring MVC):
• @RestController is a specialized form of @Controller that combines @Controller and @ResponseBody. It is often used to build RESTful web services.
@GetMapping, @PostMapping, @PutMapping, @DeleteMapping (in Spring MVC):
• These annotations are used to define HTTP methods for handling specific URL mappings in Spring MVC controllers.
@PathVariable (in Spring MVC):
• @PathVariable is used to extract values from URI templates and pass them as method parameters in Spring MVC controllers.
@ModelAttribute (in Spring MVC):
• @ModelAttribute is used to bind form data from the request to model objects in Spring MVC.
@ResponseBody (in Spring MVC):
• @ResponseBody is used to indicate that the return value of a controller method should be serialized and sent as the HTTP response body.
These are some of the most commonly used annotations in Spring. The choice of which annotations to use depends on the specific requirements of your Spring application, such as dependency injection, configuration, web request handling, and more. Spring's annotation-based configuration simplifies the development of Java applications and promotes best practices in modern software development.